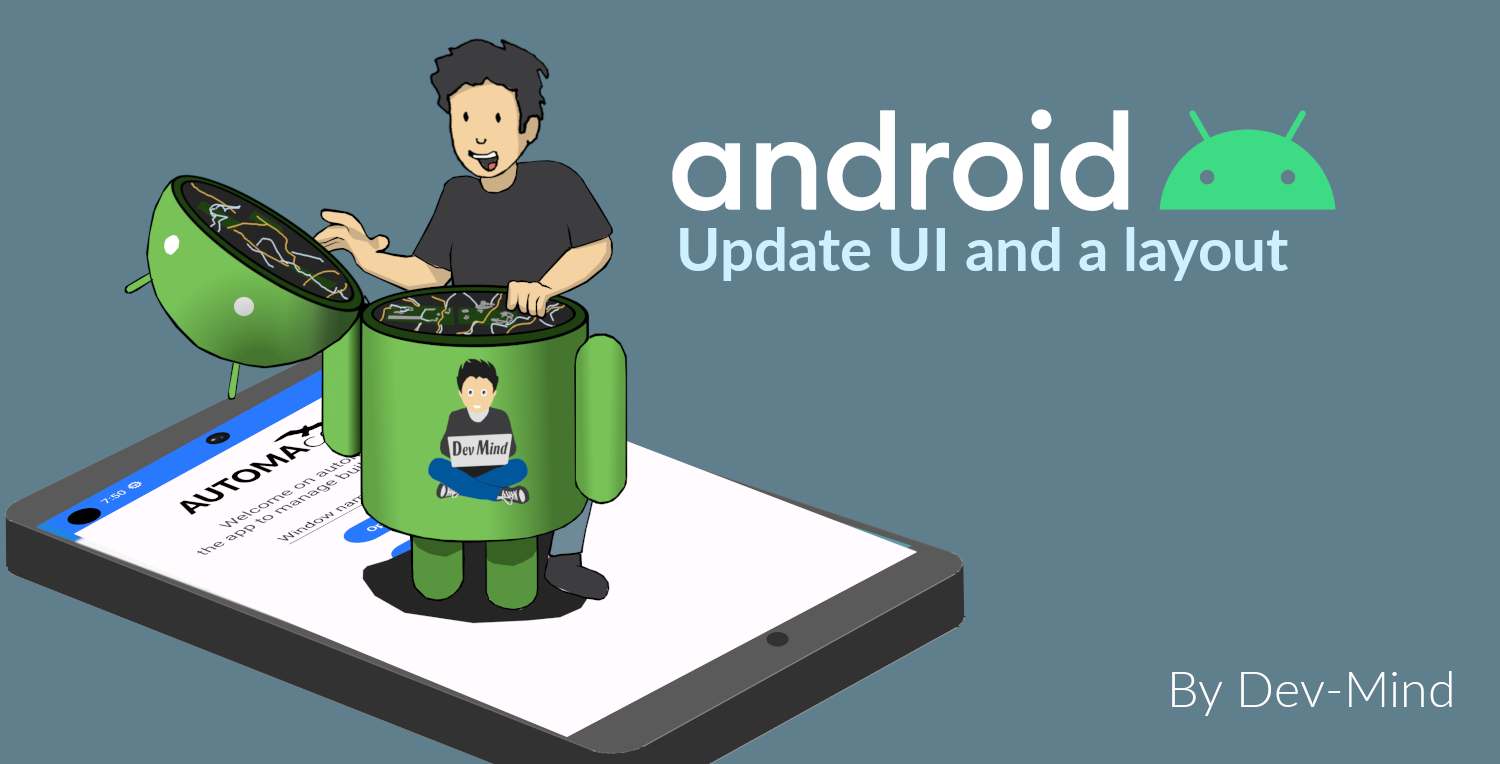
In this lesson, you will learn how to update a layout with Android Studio
User interface for an Android app is built as a hierarchy of layouts and widgets.
The layouts are ViewGroup objects, containers that control how their child views are positioned on the screen.
Widgets are View objects, UI components such as buttons and text boxes.
In this new codelab you will update the hello world
page to create a home page with a welcome message, an image, an edit text and a button.
Android provides an XML vocabulary for ViewGroup and View classes, and your UI is defined in XML files. Don’t be afraid Android Studio provide a wysiwyg editor.
In the last versions of Android, Google introduce a new way to define your UI with Jetpack Compose. With this solution you don’t need to write your templates in xml, but this technology is not yet widespread.
In the Project window, open app > res > layout > activity_main.xml. Editor should be displayed
View mode: View your layout in either code mode (XML editor), Design mode (design view and Blueprint view), or Split mode icon (mix between code and design view)
Palette: Contains various views and view groups that you can drag into your layout.
Design editor: Edit your layout in Design view, Blueprint view, or both.
Component Tree: Shows the hierarchy of components in your layout. It is sometimes useful to select a given widget
Constraint widget: Helps to place an item in relation to those around it
Attributes: Controls for the selected widget’s attributes.
Layout Toolbar: Click these buttons to configure your layout appearance in the editor and change layout attributes as target phone, orientation, light, locale…
Widget Toolbar: Click these buttons to align your view. Button with red cross is useful to clear all widget constraints
For the moment our page contains only one readonly text field.
Select it and delete it with Suppr key
We will add an image. Copy this xml file ic_logo.xml in your directory _res > drawable. This file is a vector drawable image. Directory drawable contains all your images. Several formats are available (png, jpg…) but the most optimized is a Vector drawable
In Common Palette on the left of the screen click on ImageView and drag into your layout. A window is opened to select an image. You will choose the imported image ic_logo.xml.
Click on OK button to import image in your layout
We will use the blueprint view to add constraint to this image, to place it on the top of the screen and define a height. See video below for more detail
We will add a new readonly text below image to introduce our app. In common palette select a Textview widget and drag into your layout.
In blueprint view you can add constraints to this textview
text : Welcome on automacorp the app to manage building windows
layout_width and layout_height : wrap_content
textSize: 18 sp
gravity : center
margin right and left 16dp, margin top 32dp
In text palette select a Plain Text widget (editable text view) and drag into your layout below your welcome message. This widget should have these properties
hint : Window name. This text will be displayed as long as the user has not entered anything else.
id : txt_window_name Android always generate a random name to each widget or layout. Id can be used later in your Kotlin code. It’s a good practice to use an explicit name as id
Apply a top, left margins and use constraint to place this widget below your welcome message
In common palette select a Button widget and drag into your layout below your welcome message. This button should have these properties
hint : Open window.
id : btn_open_window
Apply a top, right and left margins and use constraint use constraint to place this widget below welcome message and on the right of your plain text widget
Click on Run button to test your app (see chapter Run your app)
When something is wrong, Android Studio add a warning or an error button on the right of the editor toolbar (red flag on the top right)
Click on this button to see different problems (a window is opened on the bottom of your screen).
You can double click on an item to see the problem and have an explaination. Android studio display also a Fix button to help you to resolve problem
We will try to fix some errors. One of them is Hardcoded string "Window name", should use `@string
resource`. You added a Text Field and a text inside. As your application can be used by different people who speak different languages, you should always use text internalization mechanisms provided by Android.
Open the Project window and open file app > res > values > strings.xml. This is a string resources file, where you can specify all of your UI strings. It allows you to manage all of your UI strings in a single location, which makes them easier to find, update, and localize. For the moment you have only one text inside, your app name.
<resources>
<string name="app_name">Automacorp</string>
</resources>
You can launch Translations Editor, to add or edit text for different languages. In this lab we will use only one language. You can update this file to have a text description for our logo, and the text content for our welcome message
<resources>
<string name="app_name">automacorp</string>
<string name="app_logo_description">automacorp logo</string>
<string name="act_main_windowname_hint">Window name</string>
<string name="act_main_welcome">Welcome on automacorp,
the app to manage building windows</string>
<string name="act_main_open_window">Open window</string>
</resources>
You can now update your layout and yours components to add a string reference for image description and welcome message. To make a reference to a String you have to use the prefix @string/ followed by the string key
An activity is always associated with a layout file. In Lab 2 we have updated our main activity layout with a logo, a welcome message and a button. In this lesson, you add some code in MainActivity to interact with this button.
In the file app > java > com.automacorp > MainActivity, add the following openWindow() method stub:
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
}
/** Called when the user taps the button */
fun openWindow(view: View) {
// Extract value filled in editext identified with txt_window_name id
val windowName = findViewById@LTEditText@GT(R.id.txt_window_name).text.toString()
// Display a message
Toast.makeText(this, "You choose $windowName", Toast.LENGTH_LONG).show()
}
}
You might see an error because Android Studio cannot resolve View, Toast classes or R. To clear errors, click the View declaration, place your cursor on it, and then press Alt+Enter, or Option+Enter on a Mac, to perform a Quick Fix. If a menu appears, select Import class. Do the same thing for Toast and R classes. R class contains a link to all ressources defined in your app.
If you have an error with txt_window_name
check your layout and update the id of your EditTextView
Return to the activity_main.xml file and select the button in the Layout Editor. In Attributes window, locate onClick property and select openWindow [MainActivity] from its drop-down list.
You can now relaunch your app,
In window name editext fill a name
Click on the button you a message should be displayed on the bottom of the screen with the window name filled
As for a web page, you can define a style theme when you develop an Android application. The main them is defined in app > manifests > AndroidManifest.xml
By default @style/Theme.automacorp
follow material design specification. You app is configured to use a style @style/Theme.automacorp
<application android:allowbackup="true" android:dataextractionrules="@xml/data_extraction_rules" android:fullbackupcontent="@xml/backup_rules" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundicon="@mipmap/ic_launcher_round" android:supportsrtl="true" android:theme="@style/Theme.automacorp" tools:targetapi="31">
</application>
This file reference
File : res > values > themes > theme.xml and
Your theme Theme.automacorp in this file. You have in reality 2 files because the Google team encourages you to adopt a normal theme and a darker theme in night mode to consume less battery. As a reminder, the lighter the colors, the more your screen consumes and the more your battery is used.
You can see here that your custom them override a Material theme Theme.MaterialComponents.DayNight.DarkActionBar
<resources xmlns:tools="http://schemas.android.com/tools">
<!-- Base application theme. -->
<style name="Base.Theme.Automacorp" parent="Theme.Material3.DayNight.NoActionBar">
<!-- Customize your light theme here. -->
<!-- <item name="colorPrimary">@color/my_light_primary</item> -->
</style>
<style name="Theme.Automacorp" parent="Base.Theme.Automacorp" />
</resources>
File : res > values > colors.xml
Ce fichier référence les couleurs utilisées dans votre application
<?xml version="1.0" encoding="utf-8"?>
<resources>
<color name="black">#FF000000</color>
<color name="white">#FFFFFFFF</color>
</resources>
The material theme is built on different colors : a primary and a secondary color. You can also override all other additional colors, but today we only will try to use our own color scheme.
The first step is the color choice. For that go on Material color tool to defined your own app color combination. You define your prmiary color and the tool is able to compute complementary color
I choose this color combination
<?xml version="1.0" encoding="utf-8"?>
<resources>
<color name="black">#FF000000</color>
<color name="white">#FFFFFFFF</color>
<color name="primary">#2979ff</color>
</resources>
And after that I can update my theme file
<resources xmlns:tools="http://schemas.android.com/tools">
<!-- Base application theme. -->
<style name="Base.Theme.Automacorp" parent="Theme.Material3.DayNight.NoActionBar">
<<item name="colorPrimary">@color/primary</item>
</style>
<style name="Theme.Automacorp" parent="Base.Theme.Automacorp" />
</resources>
Run your app to see the new app rendering